Chapter 1: Introduction & Quick Start
Contents
1.1 What is AspJpeg.NET?
AspJpeg.NET is an advanced image management component to be used in a Microsoft IIS/.NET environment.
AspJpeg.NET enables your .NET application to dynamically create high-quality thumbnails of images in just a few lines of code. An original image may be in any of the following formats: JPEG, GIF, BMP, TIFF or PNG.
AspJpeg.NET is the native .NET twin of AspJpeg, a COM component with an almost identical set of features.
1.2 Feature Summary
- Supports JPEG, GIF, BMP, TIFF and PNG formats as input.
- Source images can be opened from disk, memory, or a recordset.
- Resized images can be saved to disk, memory or an HTTP stream.
- Supports three resizing algorithms: nearest-neighbor, bilinear, and bicubic.
- Drawing and typing on top of an image. Support for TrueType and Type 1 fonts.
- Automatic word wrapping, text alignment to the left, right, center and justified, rotation.
- Picture-in-picture support.
- Cropping, flipping, rotation, sharpening, grayscale conversion.
- Compression rate of output images can be adjusted for optimal quality/file size ratio.
- EXIF and IPTC metadata extraction from JPEG images.
- Metadata preservation during resizing.
- IPTC and EXIF metadata adding and editing.
- CMYK-to-RGB conversion.
- Sepia filter.
- Read/write access to individual pixels of an image.
- PNG alpha channel support.
- PNG output, alpha channel preservation.
- GIF transparency support.
- Brightness, contrast and saturation adjustment.
- GIF output, transparency and animation preservation.
- JPEG-to-GIF conversion.
- Antialiased drawing routines.
- Perspective projection.
- Gaussian blur, edge detection, threshold, other filters.
- Chroma key effect.
- 3D surface mapping.
- BMP output.
1.3 AspJpeg.NET vs. AspJpeg: Main Differences
AspJpeg is a classic-ASP/COM component while AspJpeg.NET is a native 100%-managed-code .NET component. Both products share the exact same extensive feature set, but there are a few minor differences in the object model and functionality:
-
In AspJpeg, the main "manager" object ASPJpeg also represents an individual image. This is somewhat inconvenient as some features such as Picture-in-Picture require that Server.CreateObject be called multiple times per script.
In AspJpeg.NET, this inconvenience has been eliminated by separating the "manager" and image functions into two separate objects, JpegManager and JpegImage, respectively. Creating more than one instance of the manager object per script is no longer necessary.
-
AspJpeg creates a new image with the method ASPJpeg.New, and opens an existing image with ASPJpeg.Open. These methods have no return values.
AspJpeg.NET's equivalents are JpegManager.CreateImage and JpegImage.OpenImage, respectively. Both methods return a new instance of the JpegImage object.
-
AspJpeg has two sets of drawing routines: the old-style methods such as DrawLine, DrawCircle, etc., and new-style antialiased methods such DrawLineEx, FillEllipseEx, etc.
AspJpeg.NET offers a single and powerful set of antialiased path-based drawing routines which allow a path to be constructed using arbitrary lines and curves, and then filled, stroked or both. A path may consist of multiple disconnected subpaths. Shortcut methods such as DrawLine, DrawEllipse, FillEllipse, etc. are still available.
- AspJpeg implements the picture-in-picture functionality via two methods: DrawImage and DrawPNG. The former handles regular images while the latter images with transparency (such as PNGs with an alpha channel.) AspJpeg.NET combines these into a single method, DrawImage, which handles images of both types.
1.5 Installation
AspJpeg.NET can be installed simply by running the installer application aspjpeg_net.exe available here. Since AspJpeg.NET requires .NET 4.0 or higher, you need to make sure the application pool assigned to the AspJpeg.NET virtual directory (created by the installer) is configured accordingly:
Otherwise, the following error will occur when running any of the code samples:
AspJpeg.NET can be installed manually as well. The component consists of a single assembly, Persits.Jpeg.dll, which needs to be placed in the /Bin subdirectory of your .NET application, or the Global Assembly Cache. This DLL does not need to be registered on the server with regsvr32.
You also need to put the registration key in the .config file of your application. Registration keys and the expiration mechanism used by AspJpeg.NET are described below.
We recommend that NTFS permissions be adjusted on the \Samples directory and all subdirectories to avoid an "Access is denied" or other errors when running the code samples. Using Windows Explorer, right-click on the directory c:\Program Files\Persits Software (x86)\AspJpeg.NET\Samples, select the Security tab and give the "Everyone" account full control over this folder. Click "Advanced..." and make sure the checkbox
1.6 Expiration Mechanism
AspJpeg.NET requires a registration key even for evaluation purposes. A free 30-day evaluation key is sent to the evaluator via email. Request your key at the Download page. Once a copy of the product is purchased, a permanent registration key is sent to the customer.
Registration keys used by AspJpeg.NET are 76-character strings containing symbols from the Base64 character set. Each key has the issue and expiration date information embedded into it securely. A typical AspJpeg.NET registration key looks as follows:
Note: The AspJpeg and AspJpeg.NET keys are not compatible with each other. Do not attempt to use your existing AspJpeg key with AspJpeg.NET, or vice versa.
Once a key is obtained, it should be placed in the appSettings section of your application's .config file, as follows:
<appSettings>
<add key="AspJpeg_RegKey" value="OWc13aTJOo8s/nT9Tp...51ZpX7CC"/>
</appSettings>
...
</configuration>
Alternatively, the registration key can be specified in your code via the RegKey property of the top-level JpegManager object, as follows:
objJpeg.RegKey = "OWc13aTJOo8s/nT9Tp8krmZc7...hT/1hsJtbZkwLx51ZpX7CC";
...
The current expiration date of the key can be retrieved via the Expires property, as follows:
Response.Write( objJpeg.Expires );
If the Expires property returns 9/9/9999 it means a permanent registration key is being used. This property throws an exception if the key cannot be found, or is invalid.
1.7 Quick Start
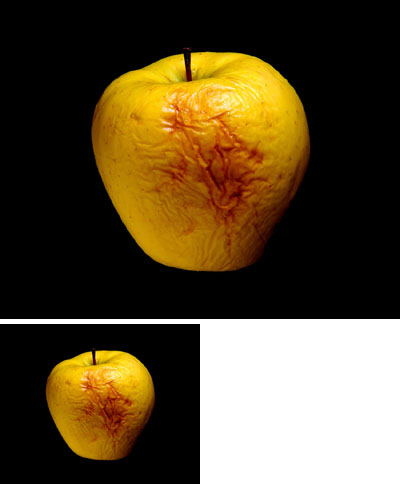
The following code sample opens an image from disk and creates a 50% scaled-down thumbnail of the image:
%@ Import Namespace="Persits.Jpeg"%>
<script runat="server" languge="C#">
void Page_Load( Object Source, EventArgs E)
{
// Create instance of JpegManager
JpegManager objJpeg = new JpegManager();
string strPath = Server.MapPath("../images/apple.jpg");
JpegImage objImage = objJpeg.OpenImage(strPath);
// Decrease image width by 50%
objImage.Width = objImage.OriginalWidth / 2;
objImage.Height = objImage.OriginalHeight / 2;
objImage.Save(Server.MapPath("apple_small.jpg"));
OriginalImage.ImageUrl = "../images/apple.jpg";
SmallImage.ImageUrl = "apple_small.jpg";
}
</script>
<form runat="server">
<asp:image runat="server" id="OriginalImage"/>
<br />
<asp:image runat="server" id="SmallImage"/>
</form>
<%@ Import Namespace="Persits.Jpeg"%>
<script runat="server" languge="VB">
Sub Page_Load( Source As Object, E As EventArgs )
' Create instance of JpegManager
Dim objJpeg As JpegManager = new JpegManager()
Dim strPath As String = Server.MapPath("../images/apple.jpg")
Dim objImage As JpegImage = objJpeg.OpenImage(strPath)
' Decrease image width by 50%
objImage.Width = objImage.OriginalWidth / 2
objImage.Height = objImage.OriginalHeight / 2
objImage.Save(Server.MapPath("apple_small.jpg"))
OriginalImage.ImageUrl = "../images/apple.jpg"
SmallImage.ImageUrl = "apple_small.jpg"
End Sub
</script>
<form runat="server">
<asp:image runat="server" id="OriginalImage"/>
<br />
<asp:image runat="server" id="SmallImage"/>
</form>
Click the links below to run this code sample:
